What are data structures in Java?
Data structures are essential components in computer science and programming. They organise and store data efficiently, enabling fast and convenient access, insertion, and deletion operations. Data structures include arrays, linked lists, stacks, queues, trees, Heaps, graphs, and hash tables.
Each data structure has its own characteristics and best use cases. Understanding data structures allows programmers to optimise algorithms and solve problems effectively by selecting the appropriate structure for a specific scenario. Mastery of data structures is crucial for efficient and scalable software development.
What is Java?
Java is a widely used, general-purpose, high-level programming language that is platform-independent and object-oriented. Java is known for its simplicity, readability, and robustness, making it a popular choice for various applications, from desktop software to web development and mobile app development.
What are Data Structures?
A data structure is a systematic and organized way of storing and managing data in a computer system. It defines the arrangement and relationships between data elements, facilitating efficient operations like storage, retrieval, and modification. Data structures provide a logical framework for organizing and accessing various types of data, such as numbers, text, and complex structures.
By choosing an appropriate data structure, programmers can optimize the performance and memory usage of their programs, enabling faster data processing and solving complex problems effectively. In essence, data structures are essential tools that enable efficient data organisation and manipulation in computer systems.
What are data structures in Java? Types of Data Structures in Java?
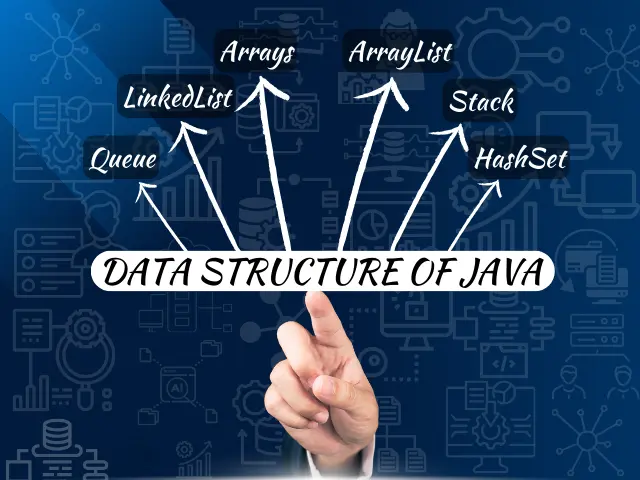
In Java, data structures are classes or interfaces that are built-in or provided through libraries to store and organize data efficiently. Here are some commonly used data structures in Java:
1. Arrays:
Arrays are fixed-size containers that store elements of the same type in contiguous memory locations.
2. ArrayList:
ArrayList is a dynamic array implementation that automatically resizes itself to accommodate elements as needed.
3. LinkedList:
LinkedList is a doubly linked list implementation where each element is stored in a node with references to the previous and next nodes.
4. Stack:
Stack is a Last-In-First-Out (LIFO) data structure that allows adding and removing elements from the top.
5. Queue:
Queue is a First-In-First-Out (FIFO) data structure that supports adding elements at the rear and removing elements from the front.
6. HashSet:
HashSet is an unordered collection that stores unique elements using a hash table implementation.
7. TreeSet:
TreeSet is an ordered set implementation that stores elements in sorted order using a tree structure.
8. HashMap:
HashMap is an unordered collection that stores key-value pairs using a hash table implementation.
9. TreeMap:
TreeMap is an ordered map implementation that stores key-value pairs in sorted order using a tree structure.
10. PriorityQueue:
PriorityQueue is a priority-based queue that orders elements based on their priority.
These are just a few examples of data structures available in Java. Each data structure has its own characteristics and use cases, and choosing the right data structure depends on the specific requirements and operations needed for the application.
Advantages of Data Structures in Java
There are several advantages of using data structures in Java. Here are some key advantages:
1. Efficient data storage and retrieval:
Data structures provide efficient storage and retrieval mechanisms, allowing for quick access to data elements. Different data structures have different access time complexities, and by choosing the appropriate data structure, you can optimize the performance of your program.
2. Enhanced data organization:
Data structures offer a systematic way to organize and structure data. They provide logical relationships between data elements, making it easier to manage and manipulate complex data sets.
3. Improved code readability and maintainability:
By using appropriate data structures, you can write code that is more readable, organized, and easier to understand. Data structures provide a clear representation of the data being used, making the code more maintainable and facilitating collaboration among developers.
4. Memory efficiency:
Data structures help in efficient memory utilization. They allocate memory based on the actual data requirements, reducing memory wastage and optimizing memory usage.
5. Increased code reusability:
Data structures provide reusable building blocks that can be used in multiple programs. By using standard data structures, you can write modular and reusable code, reducing code duplication and improving development efficiency.
6. Efficient searching and sorting:
Data structures like binary trees and hash tables offer efficient searching and sorting algorithms. These data structures provide fast lookup and retrieval of data, allowing for quick searching and sorting operations.
7. Support for algorithm design:
Data structures play a crucial role in algorithm design. Many algorithms depend on particular data structures to achieve optimal performance. By understanding and utilizing different data structures, you can design and implement efficient algorithms for various computational problems.
8. Compatibility with libraries and frameworks:
Java provides a wide range of libraries and frameworks that utilize specific data structures. By leveraging these libraries, you can take advantage of pre-built functionality and optimize your code further.
Get more details regarding Powerpoint
Phone no.
9988-500-936
Address
SCF 22, First floor, GTB Market, Khanna
Website
www.microwavecomputer.com
Opening Hours
08:30 am - 06:00 pm
FAQ
If you want to get the answer of any specific question, please feel free to contact us
Java provides several built-in data structures, including arrays, ArrayList, LinkedList, Stack, Queue, HashSet, TreeSet, HashMap, TreeMap, and PriorityQueue.
Choosing the right data structure depends on the specific requirements of your program. Consider factors such as the type of data, the operations you need to perform, the efficiency requirements, and any constraints or limitations. Understanding the characteristics and use cases of different data structures will help you make an informed decision.
Yes, you can create your custom data structures in Java. You can define classes that encapsulate the data and operations specific to your requirements. By implementing the necessary methods and functionality, you can create data structures tailored to your needs.
Java provides various methods and operations specific to each data structure. For example, you can use methods like add(), remove(), get(), push(), pop(), enqueue(), dequeue(), and more to manipulate data elements in different data structures. Consult the Java documentation or specific data structure references for detailed usage.
Java provides thread-safe implementations of certain data structures, such as ConcurrentHashMap and ConcurrentLinkedQueue, which can be safely used in concurrent multi-threaded environments. However, not all data structures in Java are inherently thread-safe. Synchronization mechanisms or concurrent data structures should be used when multiple threads access and modify the same data structure concurrently.
Some data structures in Java, such as ArrayList, HashMap, and LinkedList, are mutable, meaning their contents can be modified after creation. However, other data structures, like String and the collections provided by the Collections class (e.g., Collections.unmodifiableList()), are immutable, and their contents cannot be changed once created.
Ready to get started learning Java? Register for a free demo